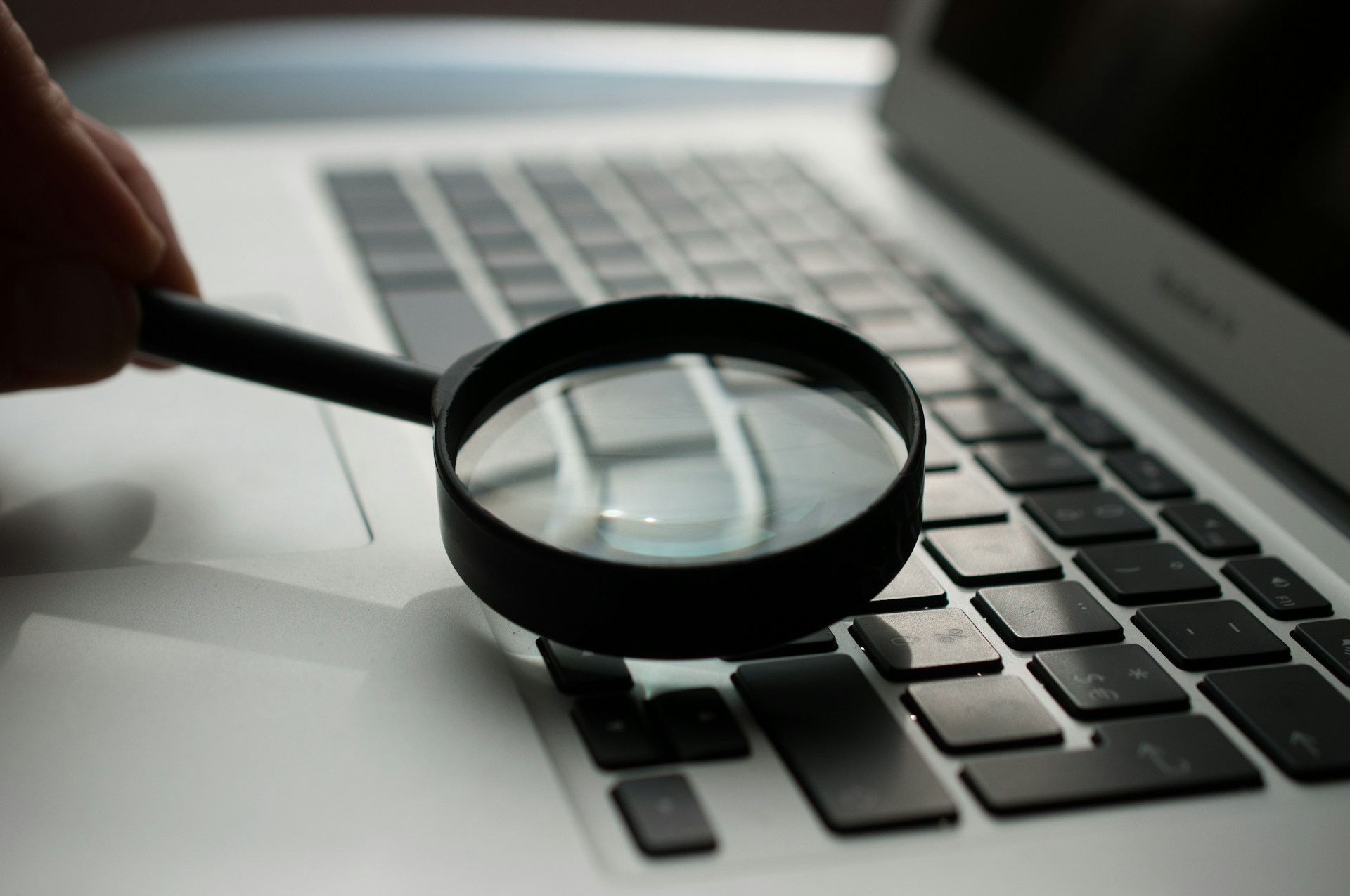
What is Binary Search?
Binary search is an efficient algorithm used to find a specific element in a sorted list. Unlike linear search, which checks every element one by one, binary search quickly narrows down the search area, significantly reducing the number of comparisons needed. This makes it much faster, especially for large datasets.
How does it work?
Start with the middle element
Split the array into two halves and check the middle element.
Compare the target value
If the middle element equals the target, you’ve found the item.
Focus on one-half
If the target is smaller, repeat the process in the left half. If it’s larger, use the right half.
Continue dividing
Keep dividing the array in half until the target is found or the search interval becomes empty.
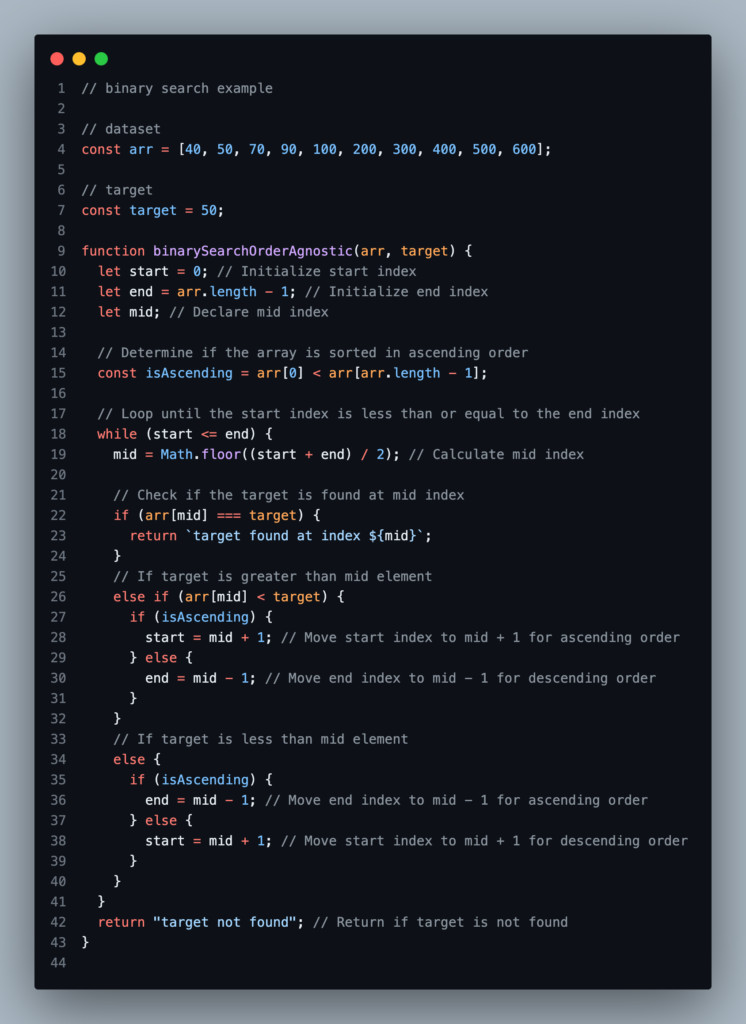
When should we use binary search?
Binary search is your go-to method when you’re dealing with a sorted list and you need to find a specific value quickly. It’s much faster than searching item by item, especially for large lists, because it splits the list in half with each step, narrowing down the search area dramatically. This makes it super efficient—think lightning-fast compared to the turtle pace of checking everything one by one. Just remember, if your list isn’t sorted yet, you’ll need to sort it first, which can take extra time. But if you’ll be searching a lot, that initial time spent sorting pays off big time in the long run.
Why should we use binary search?
Binary search is a smart choice when you’re working with a large, sorted list of items and you need to find the position of a specific value quickly. Here’s why it’s often better than just going through each item one by one:
Speed: Binary search can find an item way faster than linear search, especially as the list size grows. It cuts the list in half with each step, so it finds things in log time instead of linear time.
Efficiency: It doesn’t waste time checking every element, which saves on computing resources.
Simplicity: The algorithm is straightforward to implement and understand.
Scalability: As your list gets bigger, binary search doesn’t slow down as much as other search methods might.
Limitations
Binary search is a fast and efficient algorithm for finding an item in a sorted list, but it has some limitations:
Requires Sorted Data: Binary search only works on a sorted list of elements. If the data isn’t sorted, you’ll need to sort it first, which can be costly.
Not Optimal for Small Lists: For very small lists, the overhead of the binary search algorithm might not be worth it compared to a simple linear search.
Single-Dimensional Key Searching: It’s best suited for searching a single key in a list. For multi-dimensional searches, other algorithms might be more effective.
Poor Performance with Frequent Inserts/Deletes: If your dataset changes often, maintaining the sorted order can be expensive, reducing the overall efficiency of binary search.
Remember, despite these limitations, binary search is still a powerful tool when used under the right circumstances.
Real-world Applications
Binary search is a fast and efficient algorithm used to find the position of a target value within a sorted array. Here are some real-world applications:
Searching in Databases
When you look up a record in a large, sorted database, binary search can quickly locate the data by repeatedly dividing the search interval in half.
Computer File Systems
File systems often use binary search to locate files within directories, especially when files are sorted by name.
Optimizing Performance
In performance-sensitive applications like high-frequency trading platforms, binary search helps in making quick decisions by searching through sorted financial data.
Dictionary Lookup
Whether it’s a physical dictionary or a digital one, binary search can be used to find words quickly.
Autocomplete Features
Search engines and other autocomplete systems use binary search to predict what you’re typing by comparing it against a sorted list of possible completions.
By cutting down the search space at each step, binary search minimizes the number of comparisons, leading to faster results in these applications.
Summary
Binary search is an efficient algorithm that quickly finds a target value in a sorted array by repeatedly halving the search range. For unsorted and small datasets, linear search is more appropriate.